COMS 4733 - Lab 2, due
2/22/07 before class
Tangent Bug Path Planning
Description
(100 pts) This lab builds upon the work done in lab 1. The user will utilize MoverGUI to specify waypoints, as in lab 1,
except that when obstacles are encountered, they will be avoided utilizing the Tangent Bug algorithm.
The Tangent Bug algorithm consists of two modes:
drive towards waypoint and drive around obstacle. When the PER, using its IR rangefinder, determines that its path
towards the next waypoint is unobstructed by any obstacles, the PER will drive directly toward the obstacle. If the path is
obstructed, however, then the PER must navigate around the obstacle by driving around tangent to the surface of the obstacle, until reaching a
position where it is closer to the destination waypoint than when it became obstructed and can proceed to the designated waypoint.
Unlike in lab 1's Extra Credit, you cannot assume anything about the size the obstacle(s), specifically expect that your program will be tested with
obstacles requiring multiple movements to navigate around the obstacle. For an overview of various bug algorithms, see Siegwart 6.2.2 or the
lectures notes.
Example
Figure 1 below demonstrates a hypothetical environment and set of waypoints the PER might be asked to navigate. At first,
the PER follows the waypoints unobstructed. At the second waypoint, however, the PER realizes that it cannot proceed directly towards
the third waypoint. Therefore after having scanned the object in front of it, it then begins a path driving tangent to the wall. It continues
this process, until it reaches a position where the robot is now closer to the waypoint and IR scanning shows it can proceed directly to the next waypoint.
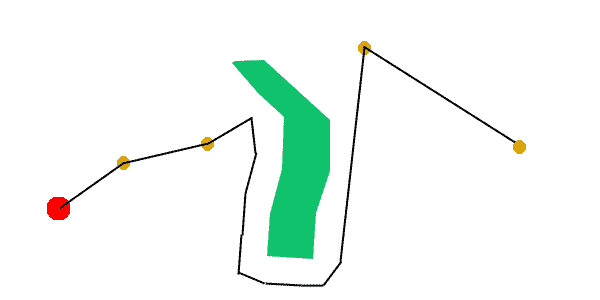
Figure 1
The program should function as follows:
- when the path to the waypoint is unobstructed, the PER should drive directly towards it.
- if the path is obstructed, the PER should determine so using its IR range finder. It should not rely upon the automatic safety features of
the DriveToAction since this often brings the PER very close to potential obstacles,
where the IR rangefinder will not give accurate results. Theoretically this could be avoided by backing up each time, but with each additional
movement, odometry errors accumulate, putting the PER further and further off-track.
- if the distance between two waypoints is farther than the maximum range of the PER's IR sensor, then the PER should make the traversal in
multiple steps, each time stopping to check that it can still proceed unobstructed.
- each "legitimate" IR scan value should be converted into the global coordinate frame and drawn within MoverGUI. Therefore a partial outline
of any obstacles encountered should be visible within MoverGUI. See the implementation of ScanAction.translateScan for bounds cases on IR values.
API Suggestions:
- DriveToAction(distance, 0, DriveToAction.NO_SAFETY, false): to drive PER
- TurnToAction(angle): to rotate PER
- TurnHeadAction(pan, tilt): to rotate PER's head when using IR rangefinder.
- ScanAction: may be useful in determining obstruction, but additional scanning will likely still be needed for mapping out obstacles.
- rov.state.getRange()/getRangeCM()/translateScan(): to read IR sensor.
Check the implementation of translateScan to see how getRangeCM functions, especially bounds conditions.
- Apache Commons Math Library: An open-source math library
containing many potentially useful functions including LSE, linear algebra, statistics, etc.
See the User Guide for an overview.
Odometry error and calibration:
It is highly recommended that you calibrate the PER, especially the pan/tilt,
else the calculated locations of the obstacles will be incorrect.
Files:
robotics_lab2.zip
To get you started, feel free to use this archive which contains the code for the demo used in class.
Note that archive is actually an export of an eclipse project, meaning it contains everything needed
to setup a completely working setup directly into eclipse (including the PER libraries and the apache math commons).
To do so, right-click the workspace tab-->import-->General-->Existing projects into workspace-->Choose robotics_lab2.zip as the archive.
Submission
- Deadline: 2/22/07 before
class starts
- What to submit: all your
source code.
Also attach a README file with
detailed compilation instructions, and
your custom Makefile (if
any). Please put all your files into an archive bearing your group's name (RedTerror_hw1.zip for
example). If you use eclipse, feel free to just export an archive file of your workspace.
- How to submit: via email with "[CS4733]-HW2" in the subject line to
dal2103@columbia.edu.
- Demoing: For this project you will be required to demo your project. E-mail the TA to arrange
a time in order to setup a time.